Excluding files and directories from a CDK Bucket Deployment
When uploading files and directories during a CDK bucket deployment, `exclude` can be used. This samples shows how to use that feature.
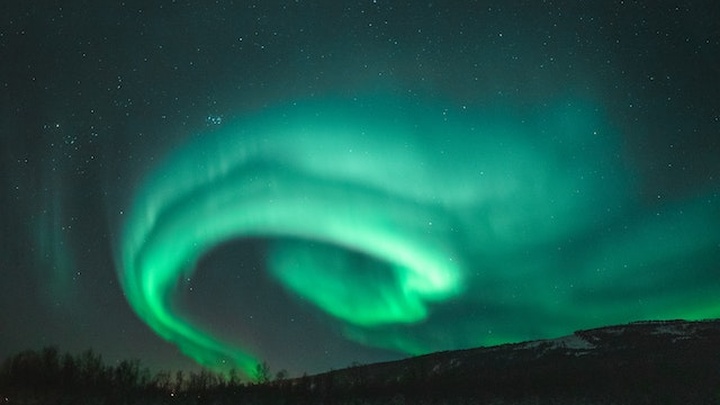
import { RemovalPolicy, IgnoreMode } from "aws-cdk-lib";
import { Bucket, ObjectOwnership } from "aws-cdk-lib/aws-s3";
import { Source, BucketDeployment } from "aws-cdk-lib/aws-s3-deployment";
import { Construct } from "constructs";
export class BucketDeploymentExample extends Construct {
constructor(scope: Construct, id: string) {
super(scope, id);
const assetBucket = new Bucket(this, "assetBucket", {
publicReadAccess: false,
removalPolicy: RemovalPolicy.DESTROY,
objectOwnership: ObjectOwnership.BUCKET_OWNER_PREFERRED,
autoDeleteObjects: true,
});
new BucketDeployment(this, "assetBucketDeployment", {
sources: [
Source.asset("src/resources/server/assets", {
exclude: ["**/node_modules/**", "**/dist/**"],
ignoreMode: IgnoreMode.GIT,
}),
],
destinationBucket: assetBucket,
retainOnDelete: false,
memoryLimit: 512,
});
}
}
Here we can see an example of how to prevent some files and directories from being uploaded as part of the bucket deployment. In this case, we want to prevent **/node_modules/**
and **/dist/**
from being uploaded as they are part of the local development and not needed in the bucket. We are going to use IgnoreMode
to use the .gitignore specification
.
Now, when using BucketDeployment
with a Source
, we can use AssetOptions
to prevent those entire directories from being included in the upload.